How to make HTTP Requests in Javascript
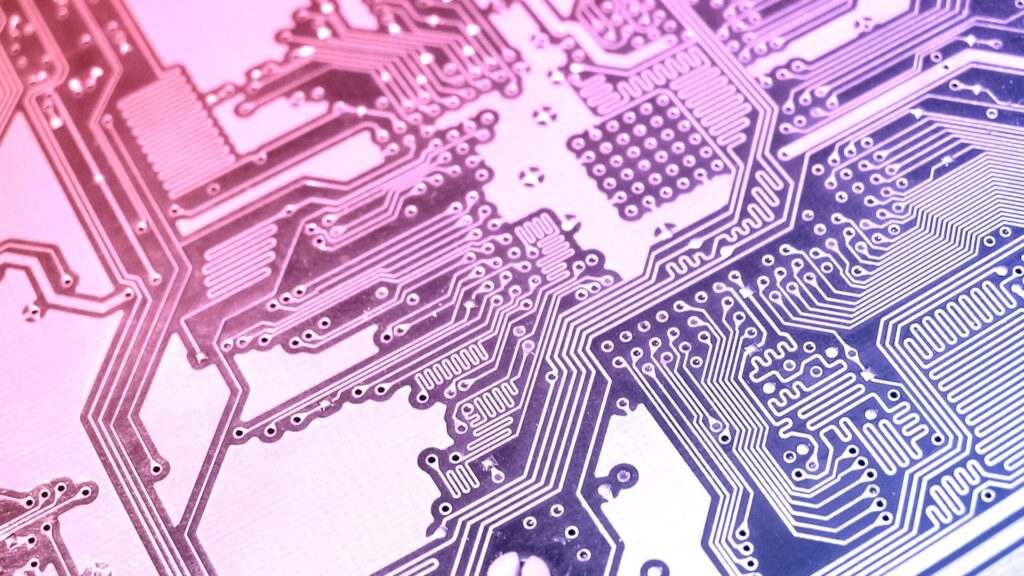
To make an HTTP request in JavaScript, you can use the XMLHttpRequest
object or the newer fetch()
API.
Here’s an example using the XMLHttpRequest
object:
javascriptCopy codelet xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
let response = xhr.responseText;
console.log(response);
}
};
xhr.send();
In this example, we create a new XMLHttpRequest
object and call its open()
method to specify the HTTP method (GET
), URL (https://example.com/api/data
), and whether the request should be asynchronous (true
).
We then set the onreadystatechange
property to a function that will be called each time the state of the request changes. In this function, we check that the request is in the readyState
of 4
(indicating that the response is complete) and that the status
is 200
(indicating a successful response). If these conditions are met, we can access the response body using the responseText
property.
Finally, we call the send()
method to send the request.
Alternatively, here’s an example using the fetch()
API:
javascriptCopy codefetch('https://example.com/api/data')
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we call the fetch()
method with the URL of the API endpoint. This returns a Promise
object that resolves to a Response
object.
We then chain two then()
methods to this Promise
. The first then()
method converts the response body to text using the text()
method. The second then()
method logs the text data to the console.
If there’s an error, the catch()
method will log the error to the console.
The fetch()
API provides a more modern and concise way of making HTTP requests in JavaScript, and it also supports async/await syntax for even more readable code.